Congratulations! You’re just about ready to jump into the development of your application! But before you get started writing code, there are a few preliminary decisions and management tasks you’ll need to take care of. In this lesson, we’ll be covering how to choose your tech stack as well as best practices for setting up your project.
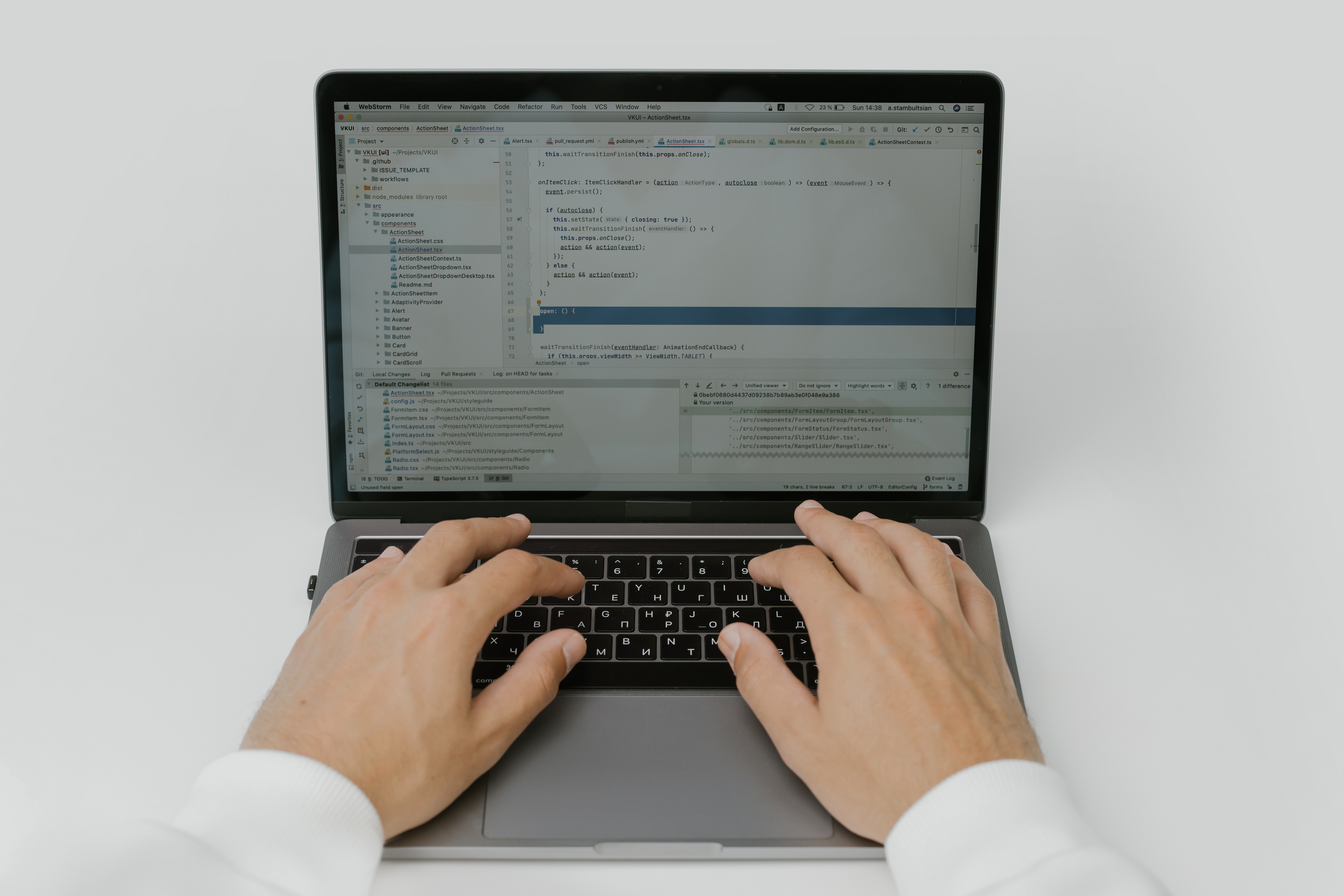
Choosing Your Tech Stack
Choosing your base tech stack is one of the critical decisions you’ll need to make at the very beginning of your project. This step is not one to be taken lightly as your decision will have a multitude of ramifications - not just for the entirety of your project, but for the lifetime of your product.
Your base tech stack includes the primary programming languages, frameworks, and libraries that will make up the core foundation of your product. There are a lot of options available for you to choose from, but it’s important that you do your research before jumping into a decision.
While there will inevitably be parts of your application you’ll need to re-write in something other than your primary language, you’ll definitely want to avoid the costly and time-consuming prospect of re-writing your entire application should you realize you’ve made a hasty decision and chose the wrong framework/language for your application.
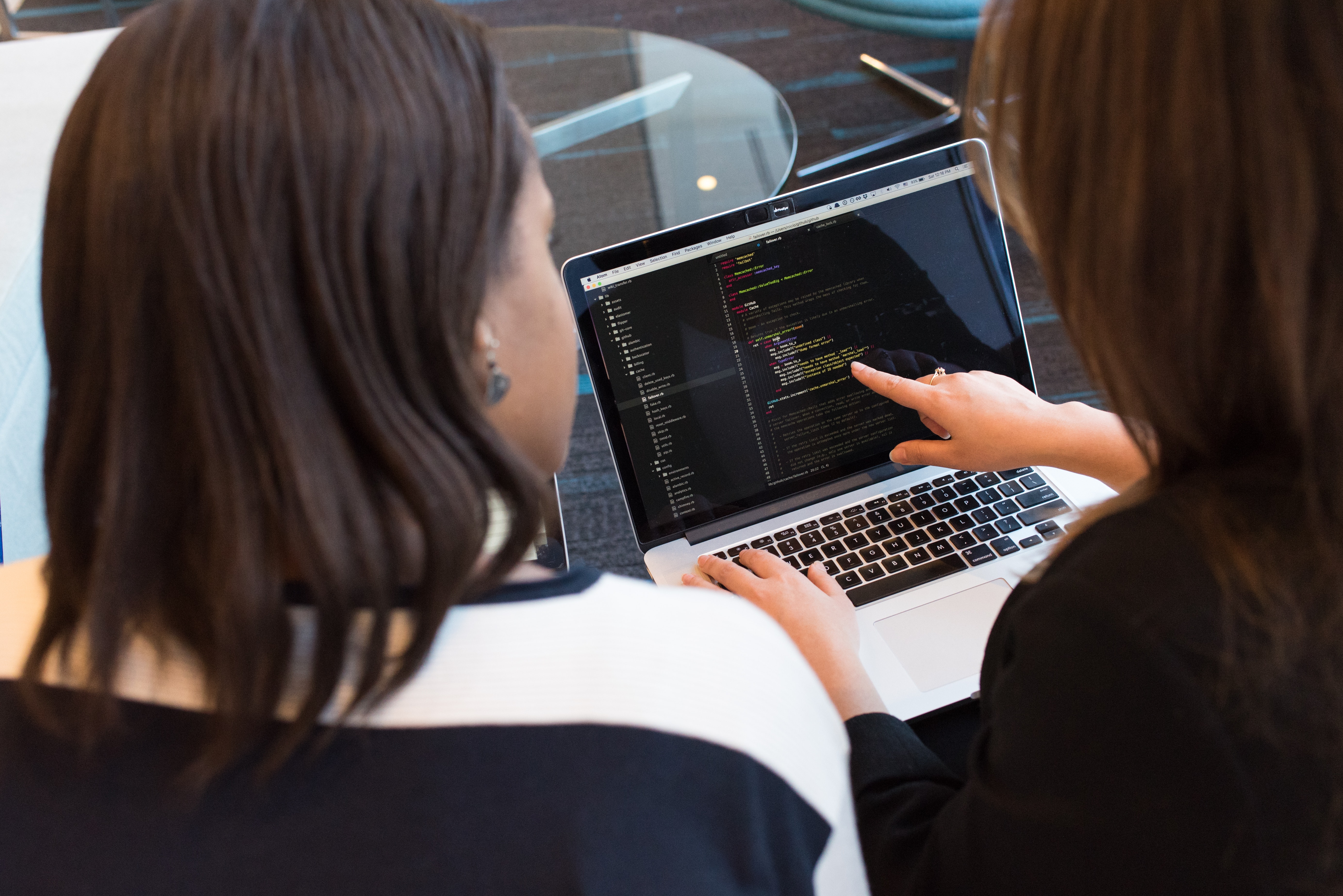
How do you Choose Your Tech Stack?
When choosing your tech stack make sure to as yourself the following three questions:
1. Who are the engineers working on your product?
It’s important to consider the skill sets and strengths of the engineers working on your team. If, for example, your engineers are great with Python, then working with Python could be the best decision - as long as it fits with the rest of the needs in this list.
If you are thinking of using technologies that are unfamiliar to your team, it’s important to consider the time, effort, and costs associated with this choice. It may go without saying that a senior engineer will have the ability to pick up a new programming language/framework/technology with more ease and speed than a Junior engineer, who will likely spend a good portion of the initial part of your project trying to learn the framework by studying examples and reading documentation.
Before choosing your framework you should always consult with your team to determine whether they feel confident that they can build what you need within your specified timeline, given the technologies you’re choosing.

2. How long has the framework been around?
Do your research and take into account how established the frameworks you are considering using are. Have they been around forever, or are they on the cutting edge - or somewhere in between?
While many engineers enjoy being on the cutting edge and utilizing the latest frameworks to emerge, consider the fact that very new technologies often have bugs, unclear best practices, and a smaller community surrounding it.
The issue of community size is especially relevant to the speed and ease with which you’ll be able to complete your application. Frameworks that are used by a large number of developers will undoubtedly have many 3rd party tools and open source libraries that your team can utilize, saving you a ton of time and effort.
Additionally, frameworks in wide use will have many more resources available. Blog articles, videos, meetups, and conferences that allow developers to learn and work through problems will indirectly increase their velocity and how quickly they can build your application.

3. What Services Will You Need to Utilize?
When determining which framework you’ll be using for your base tech stack it’s important to think about the additional technologies and services you’ll need to utilize in order for your application to function. Will your app be sending text message notifications? Will you be using two-factor auth? If so, have you identified the service or technology you’re going to use to facilitate these functions?
There are many out-of-the-box services that allow you to add these functions to your app with an SDK (software development kit) that provides pre-written code that can be placed into your project. However, it’s important that you identify whether or not the out-of-the-box services you are hoping to use provide code written in the language you are using in your tech stack.
To help ensure you’re choosing the right tech stack for your application, begin by running through your product requirements, roadmap, and designs, and creating a list of everything you’ll need from a technology and services standpoint. Laying out all of these requirements and researching the services you are hoping to use will help ensure you’re choosing a tech stack that will support all of your needs.
4. What Platform(s) is Your Product Launching On?
If you’re building a web application, you might want to consider where your product will be used and if your chosen tech stack is well suited for that. Will users be using your product on desktop, mobile, and/or tablet? Will they largely just be using it on their laptops? Making sure your web frameworks and libraries are well suited to the browsers and devices will be important.
If you’re building a mobile application, you may need to consider if you choose a tech stack that involves building the applications on iOS and Android in their native programming languages or using a cross-platform framework such as React Native. Changing your mind later on in your product development process would cause a rewrite of the entire application.
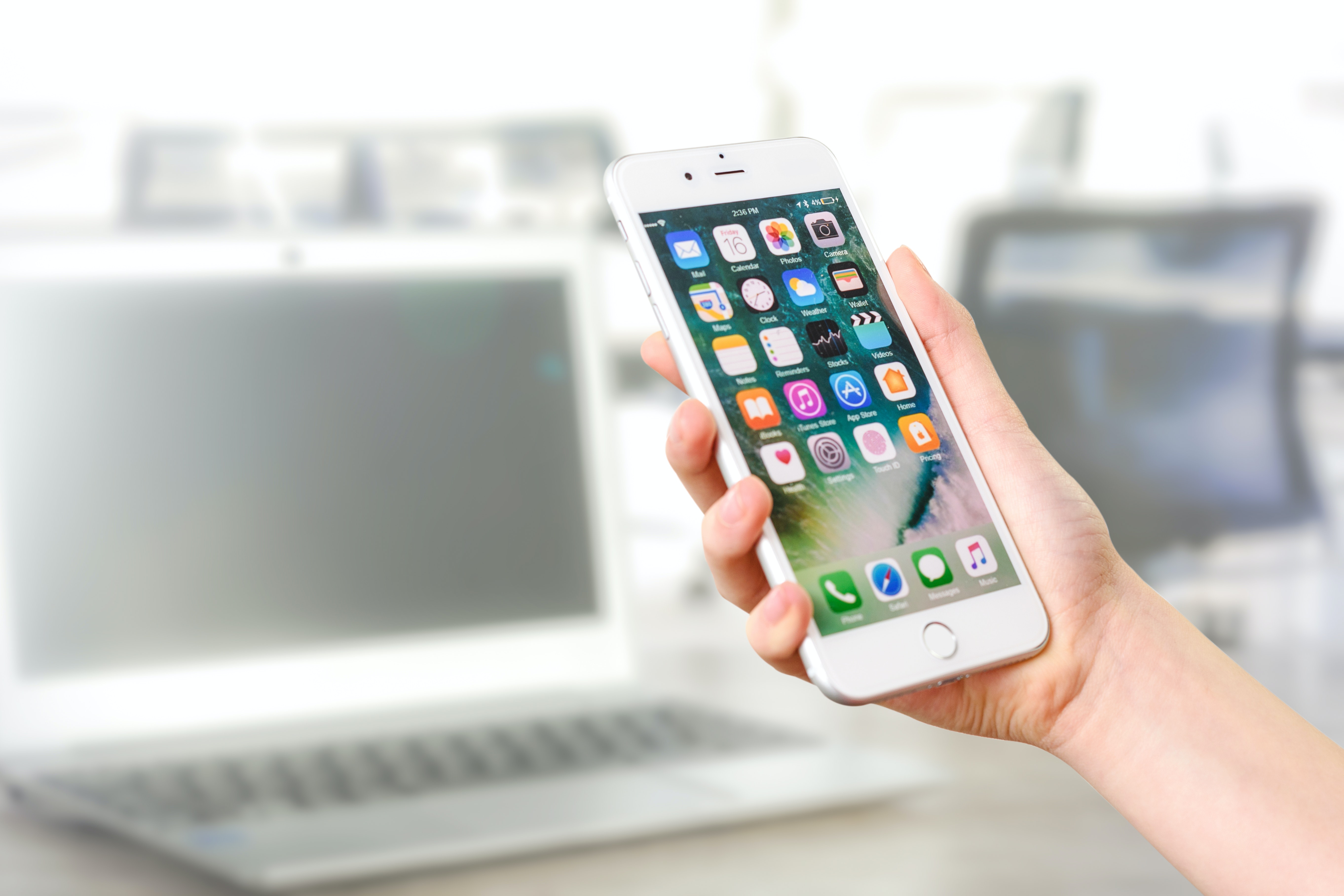
Setting up your project
Step One: Set Up Accounts
You’ll want to begin your project by setting up all the accounts you’ll need to build your application - for example, when building a mobile app, you’ll need to set up Apple and Google accounts which can actually take quite a bit of time. To prevent your team from running into any blocks down the road, you’ll also want to ensure that each developer has access to all of the accounts they’ll need to get their work done.
Step Two: Setting Up Your Codebase
Based on the decisions you’ve made about the tech stack and library you’ll be using, there may be templates that you can use to easily bootstrap up the first version of your project. These templates often allow you to turn features off and on so you can choose what you do or don’t want to include.
There are quite a few options for you to explore when looking into templates including:
Cookie Cutter: Depending on the framework you’re using, you may be able to find and use cookie-cutter templates that have been built by other developers. Many of these templates come out of the box with the ability to set up communication with your server, set up authentication, etc. These cookie-cutter templates can be extremely helpful in saving you both time and money as they save you from having to build a template from scratch.
Team Member’s Templates: It’s possible that the developers on your team might have their own templated project based on other projects they’ve worked on in the past. It’s a good idea to talk to your team and see if they have a template that can be utilized for your application.
Frameworks Utilities: Some frameworks come with a utility for setting up your app - for example, React has "Create React App"
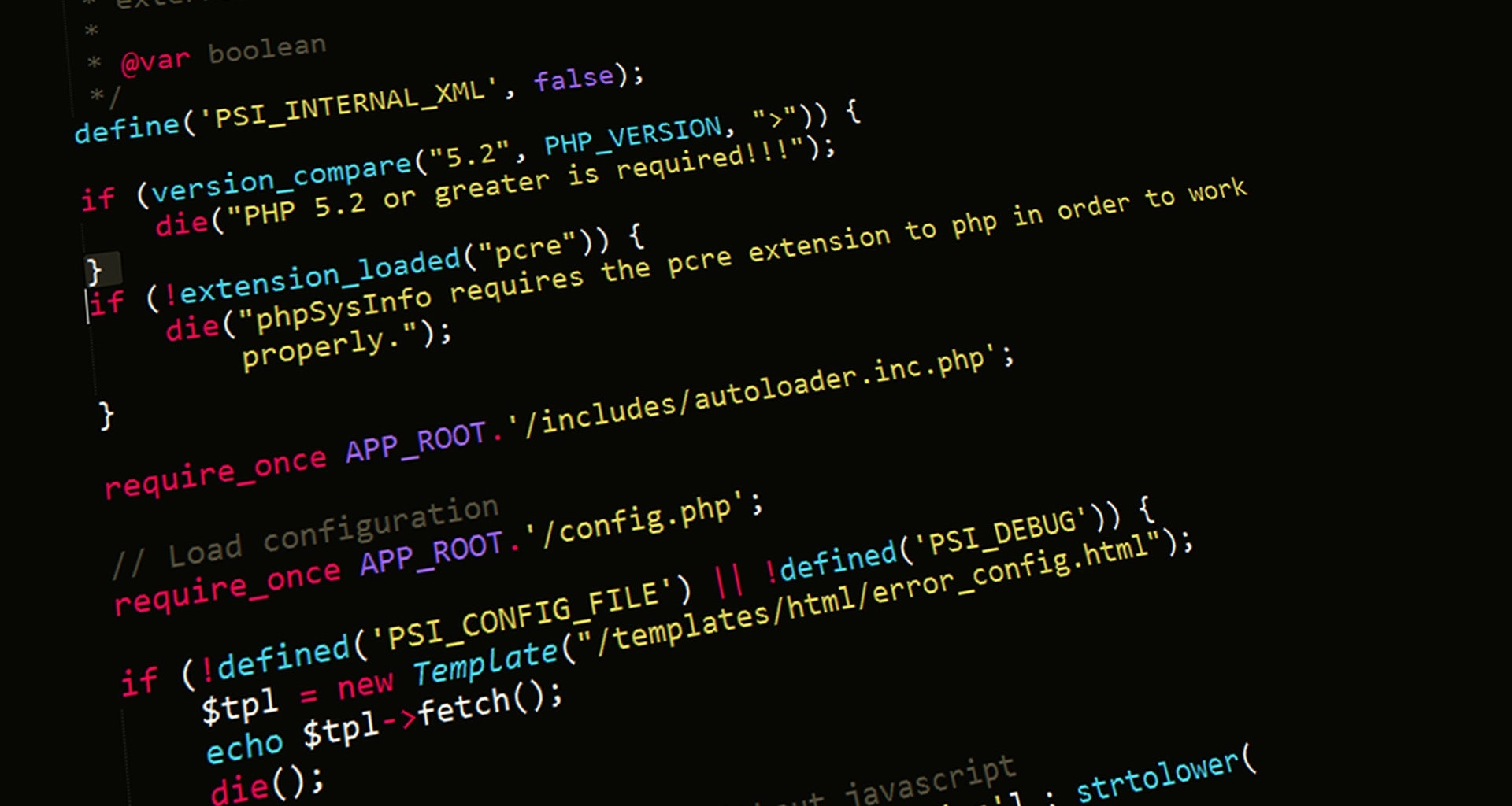
Step Three: Collaboration
It’s likely that you’ll have more than one developer working on your application and in such cases, it’s important that you set some best practices regarding how your team can best collaborate on your project.
Version Control
One non-negotiable practice your team should be employing is Version control - the practice of tracking and managing changes to software code. Utilizing version control software will be crucial to your project because if a mistake is made developers can turn back the clock and compare earlier versions of the code to help fix that mistake.
When setting up your project you should set up a version control tool like GitHub, GitLab or BitBucket. These tools will allow your developers to make changes to the code and commit those changes to the master copy. A history of each and every change and commit made will be saved, helping to ensure that everyone has the same version of the codebase and that no valuable work will ever be lost.
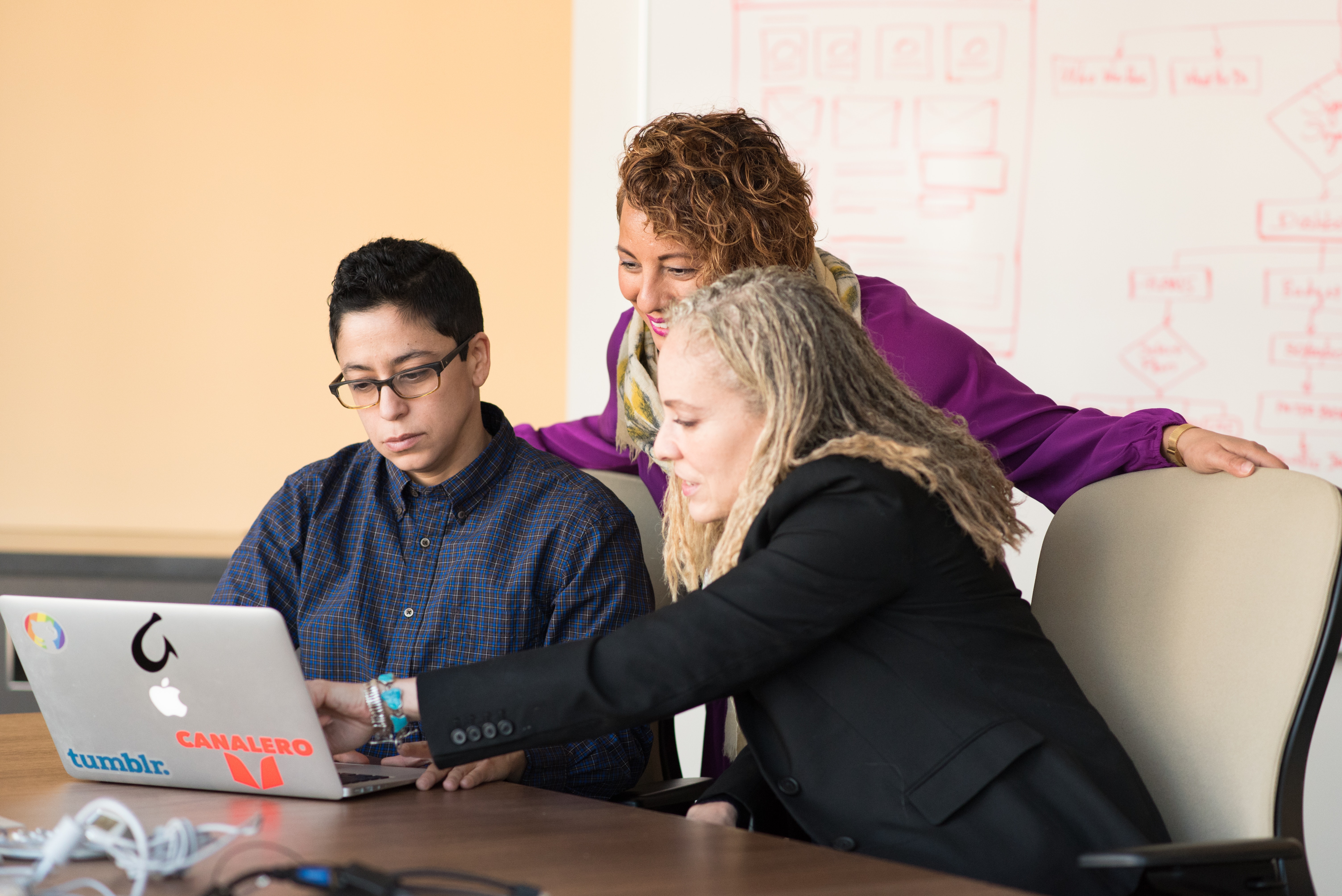
Pull Requests and Code Reviews
Oftentimes, when developers are working quickly, it may feel easiest for them to continuously make changes to the codebase and push them out non-stop. However, creating feature branches is a far better strategy. A feature branch is a copy of the main codebase where an individual or team can work on a new feature until it is complete.
Once a developer has completed their work in the feature branch they should make a pull request, in which they’ll post videos or pictures of the work they’ve done along with some notes explaining what they’ve worked on in this particular ticket. This pull request should show every line that has been deleted, edited or added.
Once a pull request has been made, other developers on the team should take a look at the pull request and do a code review, in which they ensure that everything has been done correctly. Code reviews are an especially critical practice when working with junior engineers, as senior developers can then make suggestions and catch any potential issues or bugs that might be put into the codebase.
Continuous Improvement and Continuous Deployment (CI / CD)
Throughout the lifespan of your project, there will be many instances in which other members of your team, such as designers, stakeholders, and product managers, will want to have the ability to see the latest version of your product. This can be for many reasons, including conducting user and QA testing or providing design or product feedback.
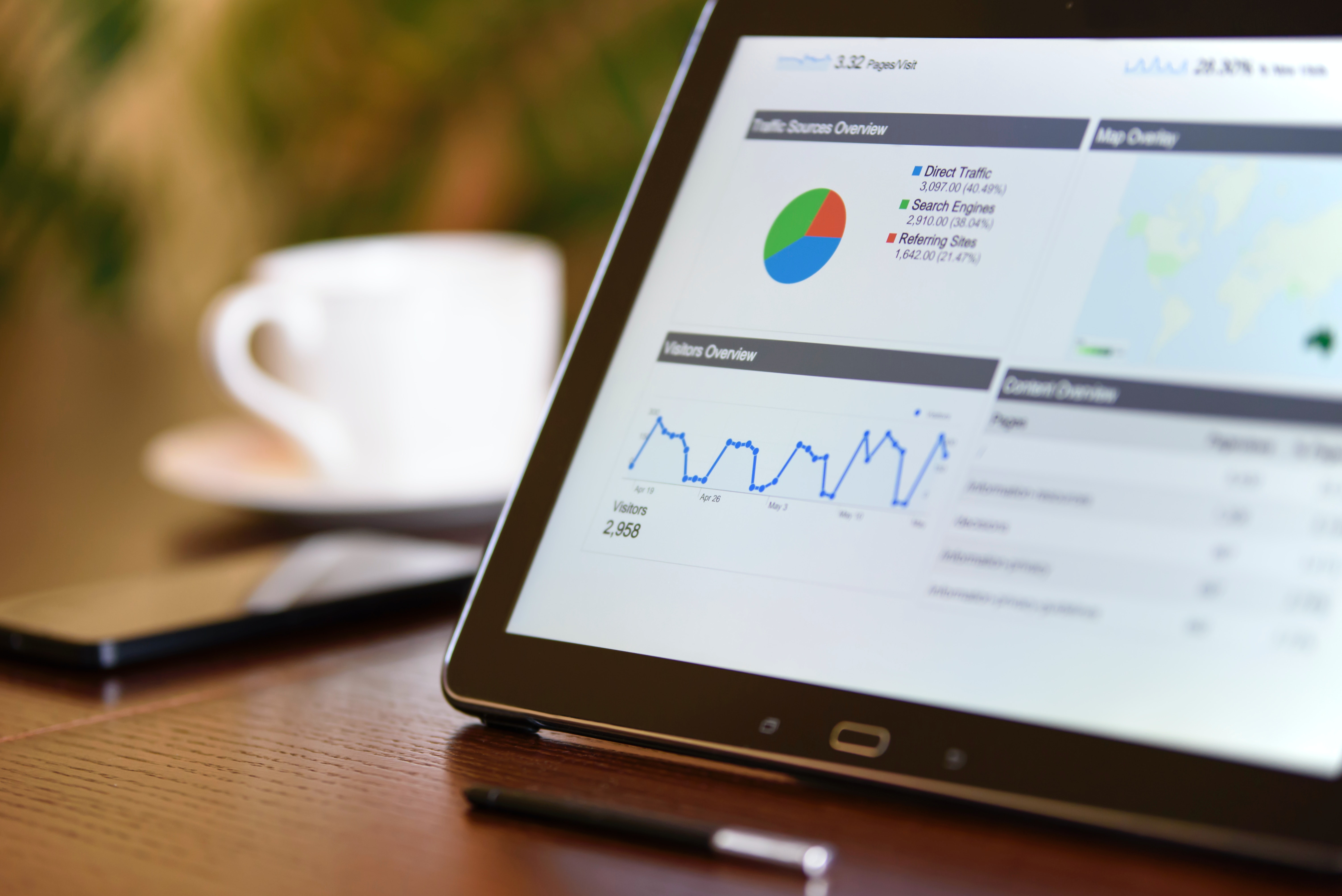
In order to avoid taking critical time away from the development team each time someone would like to view the most current version of the application, it’s best to set up a CI/CD process.
Continuous Improvement (CI) is a software development practice in which developers add their code changes to the main code branch several times a day. Each time a piece of code is added, it is automatically built and tested, providing developers with results in around 10 minutes. The purpose of this process is to produce an artifact that can be deployed and to verify that the version of code is safe to be deployed. With continuous deployment, each change in the source code is then deployed to production or a staging environment automatically, without explicit approval from a developer.
In simple terms, tools such as CircleCI and CodeShip run an automated process where the latest code is automatically deployed to a constantly running development environment that any member of the team can view.